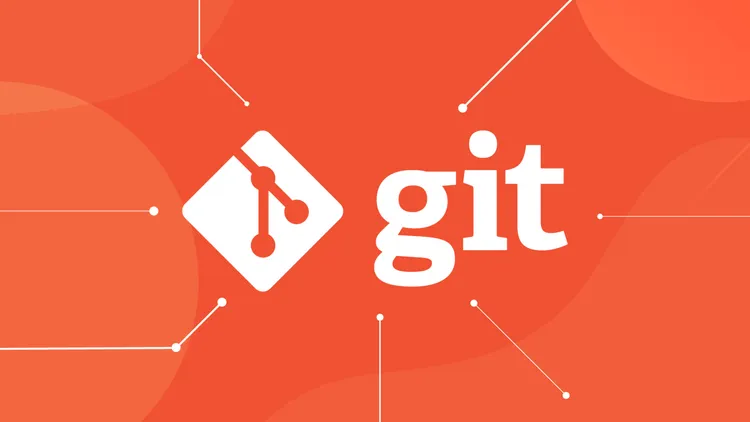
Automatically Prepend Commit Messages with Branch Names in Git
By Keshav BiswaIntroduction
If you write code in an agile environment, you probably use feature branches.
And if you use platforms like JIRA, you likely name your branches after the ticket.
Combining both practices, you might find it useful to prepend your commit messages with the branch name.
I used to manually prepend my commit message with the branch name, like this:
git commit -m "[ABC-123]: My commit message"
However, doing this manually can be tedious. So, I wrote a simple script to automate this.
Automating the Process
To automate prepending the commit message with the branch name, we’ll be using Git Hooks.
1. Create the Hook File
First, let’s navigate to our repository and create a file named prepare-commit-msg in the .git/hooks directory:
touch .git/hooks/prepare-commit-msg
2. Add the script
#!/bin/sh
branch_name=$(git symbolic-ref --short HEAD)
if [[ $branch_name == feature/* ]]; then
echo "Preparing commit message.."
# Remove the "feature/" prefix from the branch name
branch_name=${branch_name#feature/}
# Convert the branch name to uppercase (optional)
branch_name=$(echo $branch_name | tr '[:lower:]' '[:upper:]')
# Prepend the commit message with the branch name
printf "[$branch_name] %s" "$(cat $1)" > $1
echo "Commit message prepared"
fi
3. Understand the Script
Let’s break down the script and understand what it does in steps.
- The script first fetches the current branch name.
- It then checks if the branch name starts with feature/.
- If it does, the script removes the feature/ prefix. If your branch starts with a different prefix, modify this part accordingly.
- Optionally, the branch name is converted to uppercase.
- Finally, the branch name is then prepended to the commit message.
4. Make the Script Executable
For the script to work, we need to make it executable:
chmod +x .git/hooks/prepare-commit-msg
Conclusion
With this setup, every time we commit to a feature branch, our commit message will automatically be prepended with the branch name. This can save time and ensure consistency in our commit messages.